09 de fevereiro de 2023 • 5 min de leitura
The Best Way to Understand the JavaScript Map Function
Discover how to transform your data with the ease of the map function
Introduction
Have you heard of the JavaScript map
function but still don't fully understand how it works? If so, then you've come to the right place! The map
function is one of the most common operations in programming and is crucial for the development of efficient and high-performance applications.
The map function
The map
function is used to transform each element of an array into a new element. This is done by applying a specific logic or transformation to each element, resulting in a new array with the transformed elements. This operation is very useful when we need to transform raw data into processed and structured data.
The syntax of the map function consists of two main parts: the map function itself and the callback function. And this is where people usually start to get lost because the map function is a function that takes another function as a parameter, in this case the callback. But don't worry, let's understand this in a less complicated way.
The map function has the following syntax:
array.map(callback)
Where the map function is applied to an array and the callback function is executed on each element of that array.
The callback function has the following syntax:
callback(currentValue, index, array)
Where "currentValue" is the current value being processed in the array, "index" is the index of the current element being processed in the array, and "array" is the array itself being processed.
The map function returns a new array with the results of the callback function applied to each element of the original array.
Here is an example of using the map
function to increase the value of each element in an array by 1:
const numbers = [1, 2, 3, 4, 5];
const result = numbers.map(number => number + 1);
console.log(result);
// [2, 3, 4, 5, 6]
Implementing the JavaScript Map Function
The best way to really understand how the JavaScript map function works is to implement it yourself, in other words, make your own map function. This will give you a deep understanding of the functionality and concepts behind this function.
Here is the implementation of the map function:
function map(array, callback) {
const newArray = [];
for (let i = 0; i < array.length; i++) {
newArray.push(callback(array[i], i, array));
}
return newArray;
}
This implementation is simple and easy to follow, and clearly illustrates how the map function works.
The map function implementation starts with the creation of an empty array called newArray
, which will be filled with the results of the callback function applied to each element of the original array.
Next, we have a for
loop, which iterates through each element of the original array, with the number of loop repetitions equal to the size of the original array (array.length). The variable i
is used as an index to access each element of the original array. Within the loop, the callback function is executed for each element, passing three arguments: the current value of the element (array[i]
), the index of that element (i
), and the complete original array (array
).
The result of the callback function is pushed to the newArray
using the push
method. This process is repeated for each element of the original array until all elements have been processed and the for
loop is complete.
Finally, the filled newArray
is returned as the result of the map function.
The callback function is a crucial part of the map function implementation, as it is the function that determines how the array elements will be processed. You can write any type of callback function that you desire, as long as it accepts at least one argument and returns a value. The callback function can be as simple or as complex as you want, as long as its results can be used to fill the newArray
.
In summary, the map function implementation uses a for
loop to iterate through the elements of the original array, and the callback function to process each element. Together, these two components produce the new array filled with the results of the callback function applied to each element of the original array.
By writing the map function yourself, you can experiment with different types of callback functions and see how this affects the final result. Additionally, you can also add additional features to your map function implementation, which will give you an even deeper understanding of the functionality and concepts behind the function.
In summary, implementing the map function yourself is the easiest and most effective way to understand how it works and how it can be used in your code.
Practicing a little bit
Examples of transforming number arrays into object arrays
Consider the following number array:
const numbers = [1, 2, 3, 4, 5];
Using the map function, we can transform this array into an array of objects, where each object contains the current number and its double:
const doubledNumbers = numbers.map(number => {
return {
number,
double: number * 2
};
});
console.log(doubledNumbers);
// Output: [
// { number: 1, double: 2 },
// { number: 2, double: 4 },
// { number: 3, double: 6 },
// { number: 4, double: 8 },
// { number: 5, double: 10 }
// ]
Examples of transforming object arrays into string arrays
Consider the following object array:
const users = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 },
{ name: 'Jim', age: 35 }
];
Using the map
function, we can transform this array of objects into an array with just the user names:
const names = users.map(user => user.name);
console.log(names);
// Output: ['John', 'Jane', 'Jim']
Examples of transforming object arrays into function arrays
Consider the following object array:
const products = [
{ name: 'Laptop', price: 1000 },
{ name: 'Smartphone', price: 500 },
{ name: 'Tablet', price: 700 }
];
Using the map
function, we can transform this object array into an array of functions that return the price of the respective product:
const getPrices = products.map(product => {
return () => product.price;
});
console.log(getPrices[0]()); // Output: 1000
console.log(getPrices[1]()); // Output: 500
console.log(getPrices[2]()); // Output: 700
With these simple examples, you can already have an idea of how the map
function can be useful for transforming arrays in different ways. Additionally, by implementing the map
function, you learn more about how the for
loop and the callback
function work internally.
Conclusion
The map
function is an extremely versatile and powerful tool for data manipulation. The return of the function can be anything, from simple arrays to object arrays or functions. This allows for great flexibility in data structuring and transforming data from one form to another.
That's why the map
function is widely used in many programming projects, becoming a valuable skill for any programmer. By fully understanding the functioning of the map
function, programmers can take advantage of its potential to solve complex problems in a simple and efficient way.
If you have any questions or suggestions, don't hesitate to comment below, until next time.😉
Buy Me a Coffee 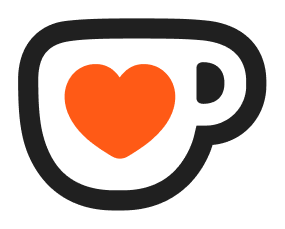
As a good programmer, I know you love a little coffee! So why don't you help me have a coffee while I produce this content for the whole community?💙
With just $3.00, you can help me, and more importantly, continue to encourage me to bring more completely free content to the whole community. You just need to click on the link below, I'm counting on your contribution 😉.
Buy Me a Coffee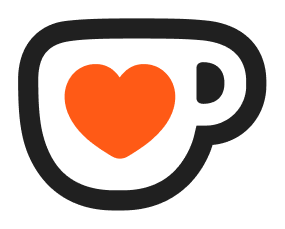
Subscribe to our Newsletter!
By subscribing to our newsletter, you will be notified every time a new post appears. Don't miss this opportunity and stay up-to-date with all the news!
Subscribe!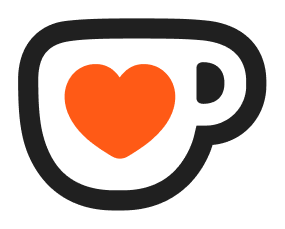